[C++ 11] 정규 표현식 std::regex (2)
https://donot-simsim.tistory.com/50
[C++ 11] 정규 표현식 std::regex (1)
https://en.cppreference.com/w/cpp/regex Regular expressions library (since C++11) - cppreference.com Regular expressions library The regular expressions library provides a class that represents regular expressions, which are a kind of mini-language used to
donot-simsim.tistory.com
정규식에 관한 간단한 문법은 위에.
C++ regex에서는 정규식을 가지고 간단하게 사용할 수 있게끔 다음 세 가지의 함수를 지원한다.
주어진 문자열이 정규식과 일치하는 [부분이 있는지] 검색하는 regex_search
주어진 문자열이 정규식과 [완벽하게] 일치하는지 검색하는 regex_match
정규식과 일치하는 부분을 다른 문자열로 변경하는. regex_replace
regex_search
해당 문자열이 정규식에 부합하는지, 아닌지를 확인하는 함수이다.
#include <iostream>
#include <regex>
using namespace std;
int main()
{
std::regex rg(R"([01]{3}-\d{3,4}-\d{4})");
auto strList = { "notmatch.txt", "010-1111-2232", "123-1-4444"};
for (auto str : strList) {
std::cout << str << ": ?" << std::endl;
if (std::regex_search(str, rg)) {
std::cout << "true" << std::endl;
}
else {
std::cout << "false" << std::endl;
}
}
return 0;
}

이런 식으로 regex_search(문자열, 정규식) 으로 간단하게 사용 가능하다.
#include <iostream>
#include <regex>
using namespace std;
int main()
{
std::regex rg(R"([01]{3}-\d{3,4}-\d{4})");
std::vector<std::string> strList = { "notmatch.txt", "010-1111-2232", "aaaa123-1-4444", "aaaa111-222-4444end" };
std::smatch matchStr;
for (auto str : strList) {
if (std::regex_search(str, matchStr, rg)) {
std::cout << str << " matched" << std::endl;
std::cout << "match str : "<< matchStr.str() << std::endl;
std::cout << "match position : "<< matchStr.position() << std::endl;
std::cout << "match prefix : "<< matchStr.prefix() << std::endl;
std::cout << "match suffix : "<< matchStr.suffix() << std::endl;
}
}
return 0;
}
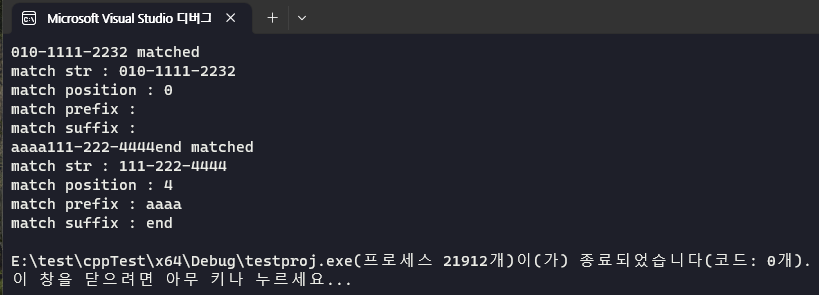
또한 이런 식으로 smatch를 사용하여 매칭 된 것들을 가지고 여러 가지를 할 수도 있다.
만약 한 줄에서 일치하는 모든 단어들을 찾고 싶다면, regex_search 대신 sregex_iterator 를 사용하면 된다.
#include <iostream>
#include <regex>
using namespace std;
int main()
{
std::regex rg(R"(\.\w{3})");
std::string input_str = "test1.txt and test2.doc is ignored";
std::smatch matched_str;
std::sregex_iterator iter(input_str.begin(), input_str.end(), rg);
std::sregex_iterator end;
while (iter != end) {
std::smatch match = *iter;
std::cout << match.str() << std::endl;
++iter;
}
return 0;
}

이렇게!
regex_match
#include <iostream>
#include <regex>
using namespace std;
int main()
{
std::regex rg(R"([01]{3}-\d{3,4}-\d{4})");
std::vector<string> strList = { "notmatch.txt", "010-1111-2232", "123-1-4444", "aaaa010-1111-2232end" };
for (auto str : strList) {
std::cout << str << ": ?" << std::endl;
if (std::regex_match(str, rg)) {
std::cout << "true" << std::endl;
}
else {
std::cout << "false" << std::endl;
}
}
return 0;
}

이런 식으로 위의 regex_search와 비슷하게 사용 가능하다.
여기서 regex_search 와 regex_match 의 차이점이라면, regex_match는 주어진 정규식과 완벽하게 매치해야만 true가 된다는 점이다.
상황에 따라 맞게 사용하도록 하자.
#include <iostream>
#include <regex>
using namespace std;
int main()
{
std::regex rg(R"([01]{3}-\d{3,4}-\d{4})");
std::vector<std::string> strList = { "notmatch.txt", "010-1111-2232", "aaaa123-1-4444", "aaaa111-222-4444end" };
std::smatch matchStr;
for (auto str : strList) {
if (std::regex_match(str, matchStr, rg)) {
std::cout << str << " matched" << std::endl;
std::cout << "match str : " << matchStr.str() << std::endl;
std::cout << "match position : " << matchStr.position() << std::endl;
std::cout << "match prefix : " << matchStr.prefix() << std::endl;
std::cout << "match suffix : " << matchStr.suffix() << std::endl;
}
}
return 0;
}
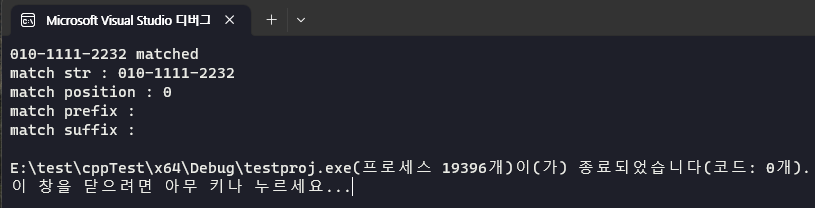
물론 regex_match도 std::smatch 를 이용하여 여러 가지 정보를 얻어올 수도 있다.
regex_replace
문자열에서 해당 문자열이 있는지 확인후, 맞는 부분이 있다면 다른 것으로 치환한다.
#include <iostream>
#include <regex>
using namespace std;
int main()
{
std::regex rg(R"(\.\w{3})");
std::string input_str = "test1.txt and test2.doc is ignored";
std::string replaced_str = std::regex_replace(input_str, rg, std::string("_someExtension"));
std::cout << replaced_str << std::endl;
return 0;
}

이런 식으로.
regex를 통해 원하는 작업을 하는 것 자체는 크게 어렵지 않다. 맞는 정규식을 쓰는 게 어렵지..
그리고 표는 봐도 봐도 헷갈리더라. 그래서 어케 항상 쓸 때마다 검색해보고 사용하는 것 같다.
그래도 요즘엔 ai가 알려주니, 검사만 한 번 해보고 바로 쓰면 되니 좋긴 하다만...